When building headless, there are generally two approaches, Static Site Generation (SSG) and Server Side Rendering (SSR). The essential difference between these two approaches is when the static content is generated. For SSG, all of the site content is turned into static files at the time the site is built. For SSR, the files are turned into static files as they're requested - once a file has been requested, it becomes a static file for all future requests (visitors) to that page.
Each approach has its own drawbacks and benefits, but I love SSG for our headless websites. They make for the most performant sites and with a framework like Astro, we can capitalize on some of the benefits of SSR not usually available with SSG (ex. Islands).
The biggest drawback with SSG is that the entire site has to be built at build time. If you have a site with a couple dozen pages and a few hundred blog posts, SSG builds wonderfully. However, a site with hundreds of pages, thousands of posts, and thousands of terms, can take forever to build. Almost an hour in some of our test cases. These builds are not only slow, they can cause performance issues on the server providing the content (WordPress in most of our cases) or memory issues on the server building the site (Netlify in most of our cases).
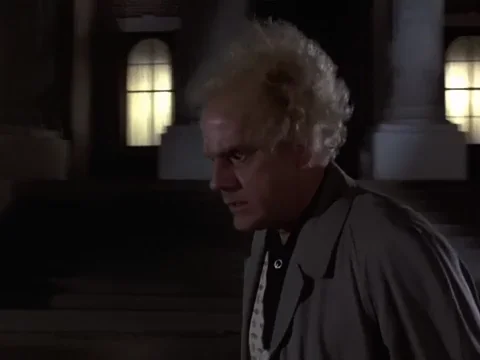
We can do better than that!
When we need to do a complete site build, there are some limited options we do have to help us improve performance. We can batch our request and cache our source endpoints (WPGraphQL Smart Cache) as well as utilize Astro data stores in the content loader.
But, this can still be a drag for content editors wanting a quick publish experience from their CMS. Getting a build that fully utilizes our cached data store in Astro while updating it with just the latest published / modified content is critical.
Getting incremental with Astro
So, for our objectives we need to:
- have a way to trigger a build from content updates within WordPress
- have builds triggered there tell our code that this is a content update
- only update modified content within our data store
- keep the ability for full data rebuilds when code updates trigger a deploy
Triggering builds within WordPress
We can trigger builds within Netlify via a webhook. When updating content, you can hook that POST request to your webhook via the save_post
action within WordPress. You could also use a plugin such as JAMstack Deployments to quickly configure you build hooks and build image URLs.
For the build hooks, Netlify does allow us to append query parameters to them to modify some of their default behavior. For our builds triggered via WordPress, I append the parameter for trigger_title
which will update how that deploy displays within our Netlify dashboard as well as be accessible within our Astro file for our content loader. So, the build hook we trigger specifically from WordPress would look like:
https://api.netlify.com/build_hooks/${hook_id}?trigger_title="wp-content-sync"
When that hook is triggered, we can access the value using the INCOMING_HOOK_TITLE
via the Astro import meta:
const INCOMING_HOOK_TITLE = import.meta.env.INCOMING_HOOK_TITLE
? import.meta.env.INCOMING_HOOK_TITLE
: "";
With this, we can conditionally modify our GraphQL requests to call just the last modified posts and not iterate our request to get all of the posts (like we would for a full site build).
const postLoader = async (after = null, results = []) => {
let hasNextPage = true;
const query = `
query GetPosts($first: Int!, $after: String, $orderby: PostObjectsConnectionOrderbyEnum! ) {
posts( where: {orderby: {field: $orderby, order: DESC}}, first: $first, after: $after ) {
pageInfo {
hasNextPage
endCursor
}
nodes {
id
title
slug
... query
}
}
}`;
const variables = {
first: 2,
after: after,
orderby: INCOMING_HOOK_TITLE != "wp-content-sync" ? "MODIFIED" : "DATE",
};
const url = "https://example.com/graphql";
try {
const response = await fetch(url, {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({ query, variables }),
});
const result = await response.json();
const posts = result.data.posts.nodes;
const pageInfo = result.data.posts.pageInfo;
hasNextPage = pageInfo.hasNextPage;
after = pageInfo.endCursor;
results.push(...posts);
if (INCOMING_HOOK_TITLE != "wp-content-sync") {
if (hasNextPage) {
return postLoader(after, results);
}
}
return results;
} catch (error) {
console.error("Error fetching posts:", error);
throw error;
}
};
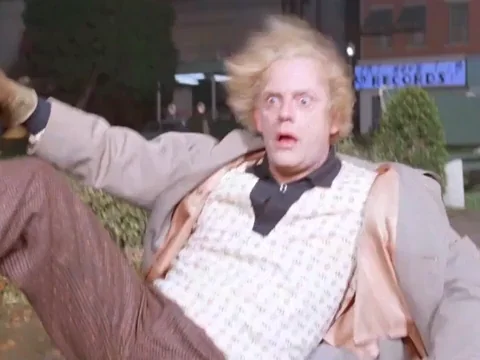
Pulling only this latest content, we can bring the build time for a site that normally take 30+ minutes to build with thousands of pieces of content plus archives down to just a couple of minutes while still maintaining full site builds when pushing code updates.